The following makes up "Assignment 0". It is intended to get you started developing right away, communicating questions/answers, and turning something in with some of the basics.
As with most assignments, a set of starter projects is available in assignment-starter/homesales-starter
.
It is expected that you can implement the complete assignment on your own.
However, the Maven poms and the portions unrelated to the assignment focus are commonly provided for reference to keep the focus on each assignment part.
Your submission should not be a direct edit/hand-in of the starters.
Your submission should — at a minimum:
-
use your own Maven groupIds
-
change the "starter" portion of the provided groupId to a name unique to you
Change: <groupId>info.ejava-student.starter.assignments.projectName</groupId> To: <groupId>info.ejava-student.[your-value].assignments.projectName</groupId>
-
-
use your own Maven descriptive name
-
change the "Starter" portion of the provided name to a name unique to you
Change: <name>Starter::Assignments::ProjectName</name> To: <name>[Your Value]::Assignments::ProjectName</name>
-
-
use your own Java package names
-
change the "starter" portion of the provided package name to a name unique to you
Change: package info.ejava_student.starter.assignment1.app.build; To: package info.ejava_student.[your_value].assignment1.app.build;
-
-
extend either
spring-boot-starter-parent
orejava-build-parent
The following diagram depicts the 3 modules (parent, javaapp, and bootapp) you will turn in. You will inherit or depend on external artifacts that will be supplied via Maven.
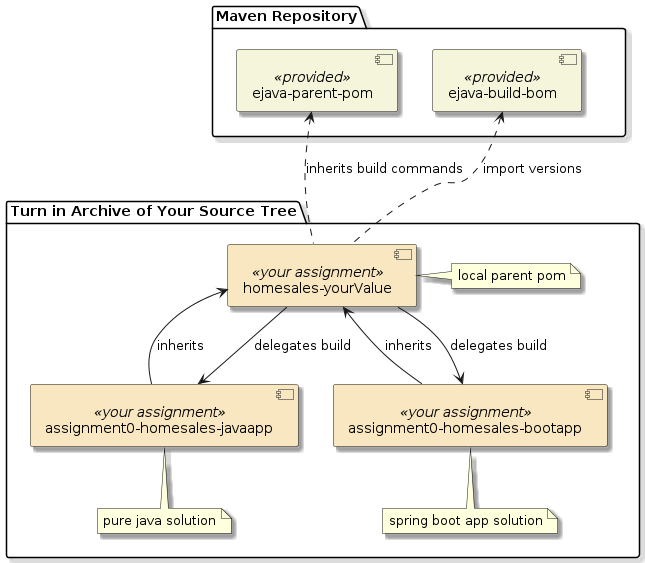
1. Part A: Build Pure Java Application JAR
1.1. Purpose
In this portion of the assignment, you will demonstrate your knowledge of building a module containing a pure Java application. You will:
-
create source code for an executable Java class
-
add that Java class to a Maven module
-
build the module using a Maven pom.xml
-
execute the application using a classpath
-
configure the application as an executable JAR
-
execute an application packaged as an executable JAR
1.2. Overview
In this portion of the assignment you are going to implement a JAR with a Java main class and execute it.
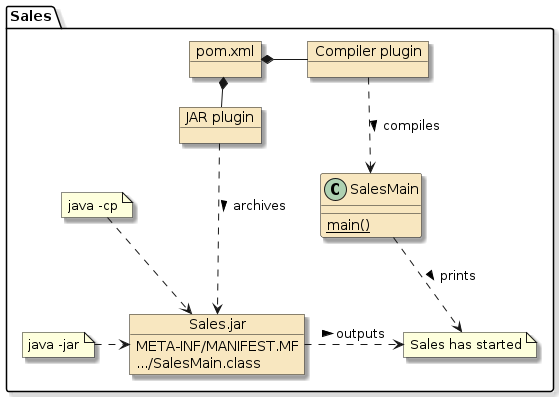
1.3. Requirements
-
Create a Maven project that will host a Java program
-
Supply a single Java class with a main() method that will print a single "Sales has started" message to stdout
-
Compile the Java class
-
Archive the Java class into a JAR
-
Execute the Java class using the JAR as a classpath
-
Register the Java class as the
Main-Class
in theMETA-INF/MANIFEST.MF
file of the JAR -
Execute the JAR to launch the Java class
-
Turn in a source tree with a complete Maven module that will build and execute a demonstration of the pure Java main application.
1.4. Grading
Your solution will be evaluated on:
-
create source code for an executable Java class
-
whether the Java class includes a non-root Java package
-
the assignment of a unique Java package for your work
-
whether you have successfully provided a main method that prints a startup message
-
-
add that Java class to a Maven module
-
the assignment of a unique groupId relative to your work
-
whether it follows standard, basic Maven src/main directory structure
-
-
build the module using a Maven pom.xml
-
whether the module builds from the command line
-
-
execute the application using a classpath
-
if the Java main class executes using a
java -cp
approach -
if the demonstration of execution is performed as part of the Maven build
-
-
execute an application packaged as an executable JAR
-
if the java main class executes using a
java -jar
approach -
if the demonstration of execution is performed as part of the Maven build
-
1.5. Additional Details
-
The root maven pom can extend either
spring-boot-starter-parent
orejava-build-parent
. Add<relativeParent/>
tag to parent reference to indicate an orphan project if doing so. -
When inheriting or depending on
ejava
class modules, include a JHU repository reference in your root pom.xml.<repositories> <repository> <id>ejava-nexus</id> <url>https://pika.jhuep.com/nexus/repository/ejava-snapshots</url> </repository> </repositories>
-
The maven build shall automate to demonstration of the two execution styles. You can use the
maven-antrun-plugin
or any other Maven plugin to implement this. -
A quick start project is available in
assignment-starter/homesales-starter/assignment0-homesales-javaapp
Modify Maven groupId and Java package if used.
2. Part B: Build Spring Boot Executable JAR
2.1. Purpose
In this portion of the assignment you will demonstrate your knowledge of building a simple Spring Boot Application. You will:
-
construct a basic Spring Boot application
-
define a simple Spring component and inject that into the Spring Boot application
-
build and execute an executable Spring Boot JAR
2.2. Overview
In this portion of the assignment, you are going to implement a Spring Boot executable JAR with a Spring Boot application and execute it.
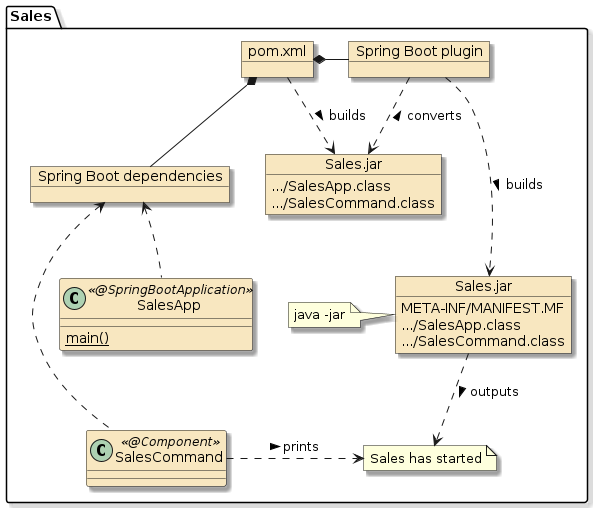
2.3. Requirements
-
Create a Maven project to host a Spring Boot Application
-
Supply a single Java class with a main() method that bootstraps the Spring Boot Application
-
Supply a
@Component
that will be loaded and invoked when the application starts-
have that
@Component
print a single "Sales has started" message to stdout
-
-
Compile the Java class
-
Archive the Java class
-
Convert the JAR into an executable Spring Boot Application JAR
-
Execute the JAR and Spring Boot Application
-
Turn in a source tree with a complete Maven module that will build and execute a demonstration of the Spring Boot application
2.4. Grading
Your solution will be evaluated on:
-
extend the standard Maven jar module packaging type to include core Spring Boot dependencies
-
whether you have added a dependency on
spring-boot-starter
to bring in required dependencies
-
-
construct a basic Spring Boot application
-
whether you have defined a proper
@SpringBootApplication
-
-
define a simple Spring component and inject that into the Spring Boot application
-
whether you have successfully injected a
@Component
that prints a startup message
-
-
build and execute an executable Spring Boot JAR
-
whether you have configured the Spring Boot plugin to build an executable JAR
-
if the demonstration of execution is performed as part of the Maven build
-
2.5. Additional Details
-
The root maven pom can extend either
spring-boot-starter-parent
orejava-build-parent
. Add<relativeParent/>
tag to parent reference to indicate an orphan project if doing so. -
When inheriting or depending on
ejava
class modules, include a JHU repository reference in your root pom.xml.<repositories> <repository> <id>ejava-nexus</id> <url>https://pika.jhuep.com/nexus/repository/ejava-snapshots</url> </repository> </repositories>
-
The maven build shall automate to demonstration of the application using the
spring-boot-maven-plugin
. There is no need for themaven-antrun-plugin
in this portion of the assignment. -
A quick start project is available in
assignment-starter/homesales-starter/assignment0-homesales-bootapp
. Modify Maven groupId and Java package if used.